728x90
반응형
docs: https://nuxt.com/docs/guide/directory-structure/pages
pages는 홈페이지의 각 페이지들이 저장됩니다. Nuxt에서 자동으로 라우팅 설정을 해주기 때문에 따로 라우팅 설정을 안해도 되는 간편함이 있습니다.
- nuxt에서 sass, scss를 사용하기 위해서 아래 명령어를 입력해줍니다.
npm install sass
- app.vue에 아래 코드를 넣어줍니다.
<template>
<nav>
<NuxtLink to="/">home</NuxtLink>
<NuxtLink to="posts">posts</NuxtLink>
<NuxtLink to="profile">profile</NuxtLink>
</nav>
<NuxtPage />
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped>
nav {
& > a {
text-decoration: none;
color: green;
&:hover {
color: blueviolet;
}
}
display: flex;
gap: 8px;
}
</style>
- NuxtLink는 라우트 해주는 컴포넌트로 to안에 원하는 경로를 입력합니다.
- script옆의 setup은 vue3에서 제공해주는 setup스크립트를 사용한다는 의미입니다. docs: https://vuejs.org/api/sfc-script-setup.html
- vue3의 setup 함수를 스크립트 전체에 적용하며 코드의 가독성과 모듈성을 매우 높여줍니다.
- style 옆의 scoped는 현재 컴포넌트에만 css를 적용한다는 의미입니다. 자세한 내용은 나중에 봅시다.
- pages 폴더 만들기 > 안에 index.vue 파일 만들기 > 아래 내용 넣기
<template>
<div class="home">
<h1>Index Page</h1>
</div>
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped></style>
- 아래와 같은 화면을 볼 수 있습니다.
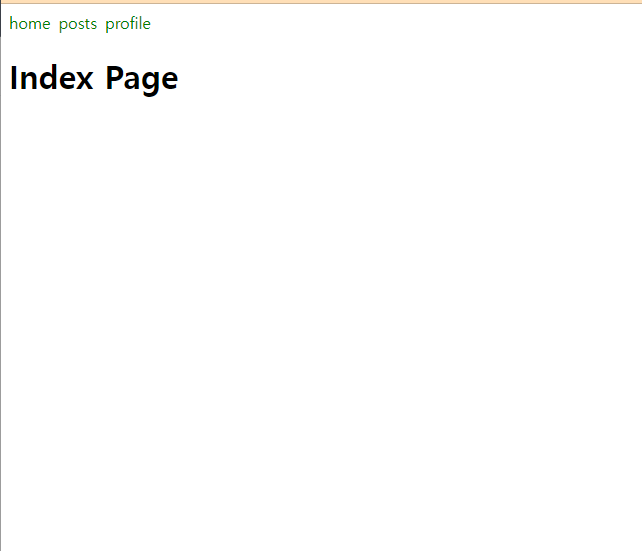
- pages 폴더 안에 profile.vue 만들기 > 아래 내용 넣기
<template>
<div class="profile">
<h1>Profile Page</h1>
<div class="profile-img"></div>
<h1>넉스트</h1>
<h2>나는 넉스트 마스터</h2>
</div>
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped>
.profile {
.profile-img {
width: 50px;
height: 50px;
border-radius: 50%;
background-color: gray;
}
}
</style>
- 이제 홈으로 가서 네비게이션의 profile 버튼을 눌러보세요. /posts/profile로 이동하는 것을 볼 수있습니다. Nuxt에서는 폴더 구조에 따라서 url을 자동으로 잡아줍니다. 겁나 편해요.
- pages 폴더 안에 posts 폴더 만들기 > 안에 index.vue 파일 만들기 > 아래 내용 넣기
<template>
<div class="posts">
<h1>Posts Page</h1>
<template v-for="post of postItems">
<div class="post" @click="$router.push(`/posts/${post.id}`)">
<h3>{{ post.id }}</h3>
<h3>{{ post.title }}</h3>
</div>
</template>
</div>
</template>
<script setup lang="ts">
interface Post {
id: number;
author: string;
title: string;
contents: string;
}
const postItems = ref<Post[]>([
{
id: 1,
author: "foo",
title: "첫번째 게시글!",
contents: "첫번째 게시글 내용!",
},
{
id: 2,
author: "foo",
title: "세상에서 제일 귀여운 고양이",
contents: "구라얌",
},
]);
</script>
<style lang="scss" scoped>
.post {
display: flex;
gap: 8px;
align-items: center;
cursor: pointer;
&:hover {
opacity: 0.8;
}
}
</style>
- posts폴더 안에 [id].vue 폴더 만들기 > 아래 내용 넣기
<template>
<div class="post">
<h1>Post</h1>
<NuxtLink to="/posts">뒤로 가기</NuxtLink>
<h2>id: {{ $route.params.id }}</h2>
</div>
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped></style>
- 결과적으로 이런 폴더 구조가 만들어집니다.
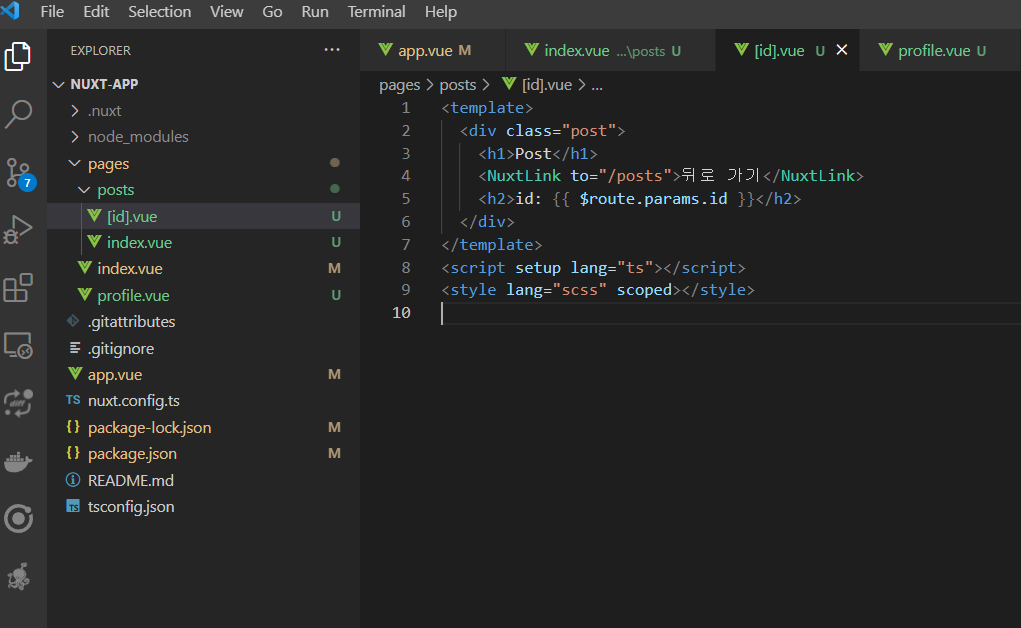
- 이제 /posts에 접속하면 포스트 리스트들을 볼 수 있고 클릭하면 각 포스트로 이동하는 것을 볼 수 있습니다. !: 혹시 보이지 않는다면 새로 고침 혹은 터미널에서 컨트롤 + c 연타 후 npm run dev를 실행해주세요.
- 무슨 원리일까요? posts 아래에 [id].vue파일을 보면 [] 안에 id라는 문자가 들어 있습니다. 이 아이디 어디서 많이 보지 않았나요?
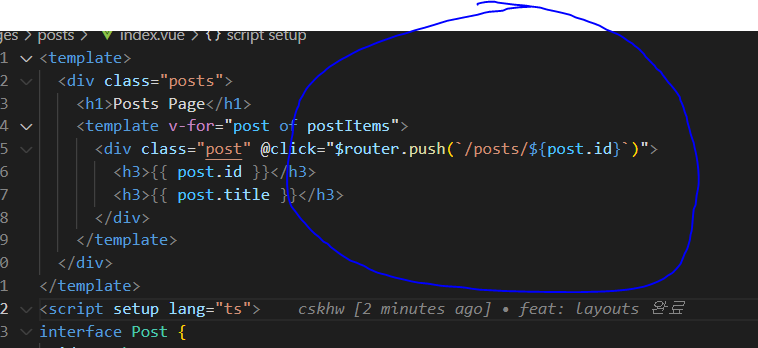
- router에서 url을 통해 id값을 전달하고 있는 것을 확인할 수 있습니다!
- 그런데 왜 id 값만 가져오고 게시글의 값은 안가져와? 라는 의문이 드실거에요. 이것은 나중에 스토어에 대해서 배워보면서 풀어 나가봅시다.
pages 끝!
pages는 홈페이지의 각 페이지들이 저장됩니다. Nuxt에서 자동으로 라우팅 설정을 해주기 때문에 따로 라우팅 설정을 안해도 되는 간편함이 있습니다.
- nuxt에서 sass, scss를 사용하기 위해서 아래 명령어를 입력해줍니다.
npm install sass
- app.vue에 아래 코드를 넣어줍니다.
<template>
<nav>
<NuxtLink to="/">home</NuxtLink>
<NuxtLink to="posts">posts</NuxtLink>
<NuxtLink to="profile">profile</NuxtLink>
</nav>
<NuxtPage />
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped>
nav {
& > a {
text-decoration: none;
color: green;
&:hover {
color: blueviolet;
}
}
display: flex;
gap: 8px;
}
</style>
- NuxtLink는 라우트 해주는 컴포넌트로 to안에 원하는 경로를 입력합니다.
- script옆의 setup은 vue3에서 제공해주는 setup스크립트를 사용한다는 의미입니다. docs: https://vuejs.org/api/sfc-script-setup.html
- vue3의 setup 함수를 스크립트 전체에 적용하며 코드의 가독성과 모듈성을 매우 높여줍니다.
- style 옆의 scoped는 현재 컴포넌트에만 css를 적용한다는 의미입니다. 자세한 내용은 나중에 봅시다.
- pages 폴더 만들기 > 안에 index.vue 파일 만들기 > 아래 내용 넣기
<template>
<div class="home">
<h1>Index Page</h1>
</div>
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped></style>
- 아래와 같은 화면을 볼 수 있습니다.
- pages 폴더 안에 profile.vue 만들기 > 아래 내용 넣기
<template>
<div class="profile">
<h1>Profile Page</h1>
<div class="profile-img"></div>
<h1>넉스트</h1>
<h2>나는 넉스트 마스터</h2>
</div>
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped>
.profile {
.profile-img {
width: 50px;
height: 50px;
border-radius: 50%;
background-color: gray;
}
}
</style>
- 이제 홈으로 가서 네비게이션의 profile 버튼을 눌러보세요. /posts/profile로 이동하는 것을 볼 수있습니다. Nuxt에서는 폴더 구조에 따라서 url을 자동으로 잡아줍니다. 겁나 편해요.
- pages 폴더 안에 posts 폴더 만들기 > 안에 index.vue 파일 만들기 > 아래 내용 넣기
<template>
<div class="posts">
<h1>Posts Page</h1>
<template v-for="post of postItems">
<div class="post" @click="$router.push(`/posts/${post.id}`)">
<h3>{{ post.id }}</h3>
<h3>{{ post.title }}</h3>
</div>
</template>
</div>
</template>
<script setup lang="ts">
interface Post {
id: number;
author: string;
title: string;
contents: string;
}
const postItems = ref<Post[]>([
{
id: 1,
author: "foo",
title: "첫번째 게시글!",
contents: "첫번째 게시글 내용!",
},
{
id: 2,
author: "foo",
title: "세상에서 제일 귀여운 고양이",
contents: "구라얌",
},
]);
</script>
<style lang="scss" scoped>
.post {
display: flex;
gap: 8px;
align-items: center;
cursor: pointer;
&:hover {
opacity: 0.8;
}
}
</style>
- posts폴더 안에 [id].vue 폴더 만들기 > 아래 내용 넣기
<template>
<div class="post">
<h1>Post</h1>
<NuxtLink to="/posts">뒤로 가기</NuxtLink>
<h2>id: {{ $route.params.id }}</h2>
</div>
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped></style>
- 결과적으로 이런 폴더 구조가 만들어집니다.
- 이제 /posts에 접속하면 포스트 리스트들을 볼 수 있고 클릭하면 각 포스트로 이동하는 것을 볼 수 있습니다. !: 혹시 보이지 않는다면 새로 고침 혹은 터미널에서 컨트롤 + c 연타 후 npm run dev를 실행해주세요.
- 무슨 원리일까요? posts 아래에 [id].vue파일을 보면 [] 안에 id라는 문자가 들어 있습니다. 이 아이디 어디서 많이 보지 않았나요?
- router에서 url을 통해 id값을 전달하고 있는 것을 확인할 수 있습니다!
- 그런데 왜 id 값만 가져오고 게시글의 값은 안가져와? 라는 의문이 드실거에요. 이것은 나중에 스토어에 대해서 배워보면서 풀어 나가봅시다.
pages 끝!
pages는 홈페이지의 각 페이지들이 저장됩니다. Nuxt에서 자동으로 라우팅 설정을 해주기 때문에 따로 라우팅 설정을 안해도 되는 간편함이 있습니다.
- nuxt에서 sass, scss를 사용하기 위해서 아래 명령어를 입력해줍니다.
npm install sass
- app.vue에 아래 코드를 넣어줍니다.
<template>
<nav>
<NuxtLink to="/">home</NuxtLink>
<NuxtLink to="posts">posts</NuxtLink>
<NuxtLink to="profile">profile</NuxtLink>
</nav>
<NuxtPage />
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped>
nav {
& > a {
text-decoration: none;
color: green;
&:hover {
color: blueviolet;
}
}
display: flex;
gap: 8px;
}
</style>
- NuxtLink는 라우트 해주는 컴포넌트로 to안에 원하는 경로를 입력합니다.
- script옆의 setup은 vue3에서 제공해주는 setup스크립트를 사용한다는 의미입니다. docs: https://vuejs.org/api/sfc-script-setup.html
- vue3의 setup 함수를 스크립트 전체에 적용하며 코드의 가독성과 모듈성을 매우 높여줍니다.
- style 옆의 scoped는 현재 컴포넌트에만 css를 적용한다는 의미입니다. 자세한 내용은 나중에 봅시다.
- pages 폴더 만들기 > 안에 index.vue 파일 만들기 > 아래 내용 넣기
<template>
<div class="home">
<h1>Index Page</h1>
</div>
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped></style>
- 아래와 같은 화면을 볼 수 있습니다.
- pages 폴더 안에 profile.vue 만들기 > 아래 내용 넣기
<template>
<div class="profile">
<h1>Profile Page</h1>
<div class="profile-img"></div>
<h1>넉스트</h1>
<h2>나는 넉스트 마스터</h2>
</div>
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped>
.profile {
.profile-img {
width: 50px;
height: 50px;
border-radius: 50%;
background-color: gray;
}
}
</style>
- 이제 홈으로 가서 네비게이션의 profile 버튼을 눌러보세요. /posts/profile로 이동하는 것을 볼 수있습니다. Nuxt에서는 폴더 구조에 따라서 url을 자동으로 잡아줍니다. 겁나 편해요.
- pages 폴더 안에 posts 폴더 만들기 > 안에 index.vue 파일 만들기 > 아래 내용 넣기
<template>
<div class="posts">
<h1>Posts Page</h1>
<template v-for="post of postItems">
<div class="post" @click="$router.push(`/posts/${post.id}`)">
<h3>{{ post.id }}</h3>
<h3>{{ post.title }}</h3>
</div>
</template>
</div>
</template>
<script setup lang="ts">
interface Post {
id: number;
author: string;
title: string;
contents: string;
}
const postItems = ref<Post[]>([
{
id: 1,
author: "foo",
title: "첫번째 게시글!",
contents: "첫번째 게시글 내용!",
},
{
id: 2,
author: "foo",
title: "세상에서 제일 귀여운 고양이",
contents: "구라얌",
},
]);
</script>
<style lang="scss" scoped>
.post {
display: flex;
gap: 8px;
align-items: center;
cursor: pointer;
&:hover {
opacity: 0.8;
}
}
</style>
- posts폴더 안에 [id].vue 폴더 만들기 > 아래 내용 넣기
<template>
<div class="post">
<h1>Post</h1>
<NuxtLink to="/posts">뒤로 가기</NuxtLink>
<h2>id: {{ $route.params.id }}</h2>
</div>
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped></style>
- 결과적으로 이런 폴더 구조가 만들어집니다.
- 이제 /posts에 접속하면 포스트 리스트들을 볼 수 있고 클릭하면 각 포스트로 이동하는 것을 볼 수 있습니다. !: 혹시 보이지 않는다면 새로 고침 혹은 터미널에서 컨트롤 + c 연타 후 npm run dev를 실행해주세요.
- 무슨 원리일까요? posts 아래에 [id].vue파일을 보면 [] 안에 id라는 문자가 들어 있습니다. 이 아이디 어디서 많이 보지 않았나요?
- router에서 url을 통해 id값을 전달하고 있는 것을 확인할 수 있습니다!
- 그런데 왜 id 값만 가져오고 게시글의 값은 안가져와? 라는 의문이 드실거에요. 이것은 나중에 스토어에 대해서 배워보면서 풀어 나가봅시다.
pages 끝!
728x90
반응형
'Leture > TodoList 만들기' 카테고리의 다른 글
728x90
반응형
docs: https://nuxt.com/docs/guide/directory-structure/pages
pages는 홈페이지의 각 페이지들이 저장됩니다. Nuxt에서 자동으로 라우팅 설정을 해주기 때문에 따로 라우팅 설정을 안해도 되는 간편함이 있습니다.
- nuxt에서 sass, scss를 사용하기 위해서 아래 명령어를 입력해줍니다.
npm install sass
- app.vue에 아래 코드를 넣어줍니다.
<template>
<nav>
<NuxtLink to="/">home</NuxtLink>
<NuxtLink to="posts">posts</NuxtLink>
<NuxtLink to="profile">profile</NuxtLink>
</nav>
<NuxtPage />
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped>
nav {
& > a {
text-decoration: none;
color: green;
&:hover {
color: blueviolet;
}
}
display: flex;
gap: 8px;
}
</style>
- NuxtLink는 라우트 해주는 컴포넌트로 to안에 원하는 경로를 입력합니다.
- script옆의 setup은 vue3에서 제공해주는 setup스크립트를 사용한다는 의미입니다. docs: https://vuejs.org/api/sfc-script-setup.html
- vue3의 setup 함수를 스크립트 전체에 적용하며 코드의 가독성과 모듈성을 매우 높여줍니다.
- style 옆의 scoped는 현재 컴포넌트에만 css를 적용한다는 의미입니다. 자세한 내용은 나중에 봅시다.
- pages 폴더 만들기 > 안에 index.vue 파일 만들기 > 아래 내용 넣기
<template>
<div class="home">
<h1>Index Page</h1>
</div>
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped></style>
- 아래와 같은 화면을 볼 수 있습니다.
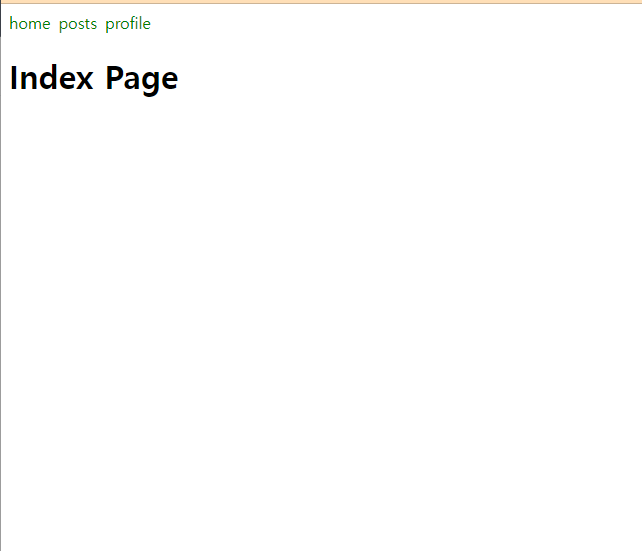
- pages 폴더 안에 profile.vue 만들기 > 아래 내용 넣기
<template>
<div class="profile">
<h1>Profile Page</h1>
<div class="profile-img"></div>
<h1>넉스트</h1>
<h2>나는 넉스트 마스터</h2>
</div>
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped>
.profile {
.profile-img {
width: 50px;
height: 50px;
border-radius: 50%;
background-color: gray;
}
}
</style>
- 이제 홈으로 가서 네비게이션의 profile 버튼을 눌러보세요. /posts/profile로 이동하는 것을 볼 수있습니다. Nuxt에서는 폴더 구조에 따라서 url을 자동으로 잡아줍니다. 겁나 편해요.
- pages 폴더 안에 posts 폴더 만들기 > 안에 index.vue 파일 만들기 > 아래 내용 넣기
<template>
<div class="posts">
<h1>Posts Page</h1>
<template v-for="post of postItems">
<div class="post" @click="$router.push(`/posts/${post.id}`)">
<h3>{{ post.id }}</h3>
<h3>{{ post.title }}</h3>
</div>
</template>
</div>
</template>
<script setup lang="ts">
interface Post {
id: number;
author: string;
title: string;
contents: string;
}
const postItems = ref<Post[]>([
{
id: 1,
author: "foo",
title: "첫번째 게시글!",
contents: "첫번째 게시글 내용!",
},
{
id: 2,
author: "foo",
title: "세상에서 제일 귀여운 고양이",
contents: "구라얌",
},
]);
</script>
<style lang="scss" scoped>
.post {
display: flex;
gap: 8px;
align-items: center;
cursor: pointer;
&:hover {
opacity: 0.8;
}
}
</style>
- posts폴더 안에 [id].vue 폴더 만들기 > 아래 내용 넣기
<template>
<div class="post">
<h1>Post</h1>
<NuxtLink to="/posts">뒤로 가기</NuxtLink>
<h2>id: {{ $route.params.id }}</h2>
</div>
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped></style>
- 결과적으로 이런 폴더 구조가 만들어집니다.
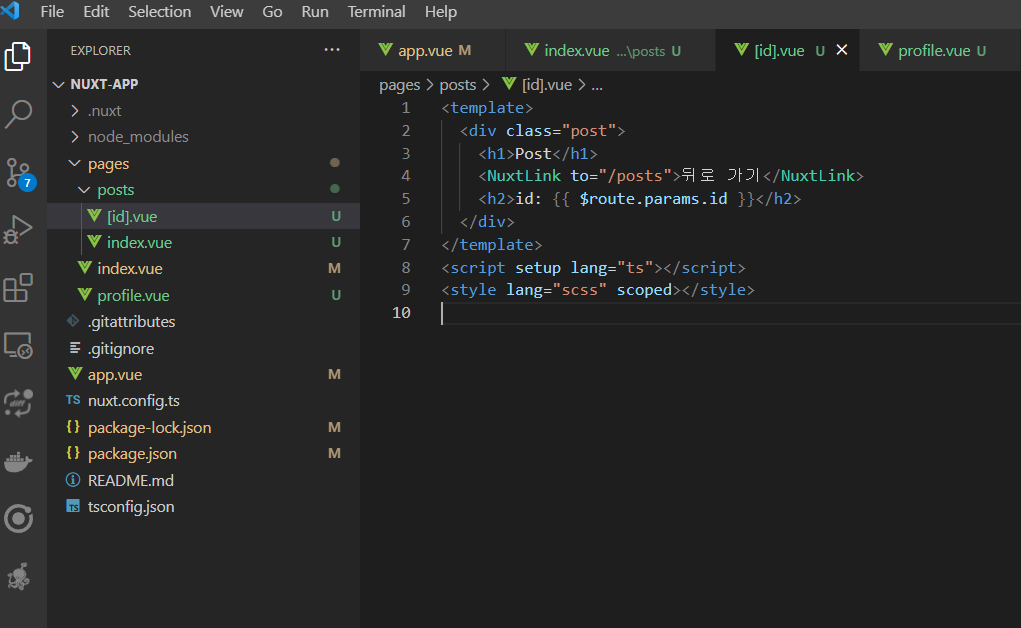
- 이제 /posts에 접속하면 포스트 리스트들을 볼 수 있고 클릭하면 각 포스트로 이동하는 것을 볼 수 있습니다. !: 혹시 보이지 않는다면 새로 고침 혹은 터미널에서 컨트롤 + c 연타 후 npm run dev를 실행해주세요.
- 무슨 원리일까요? posts 아래에 [id].vue파일을 보면 [] 안에 id라는 문자가 들어 있습니다. 이 아이디 어디서 많이 보지 않았나요?
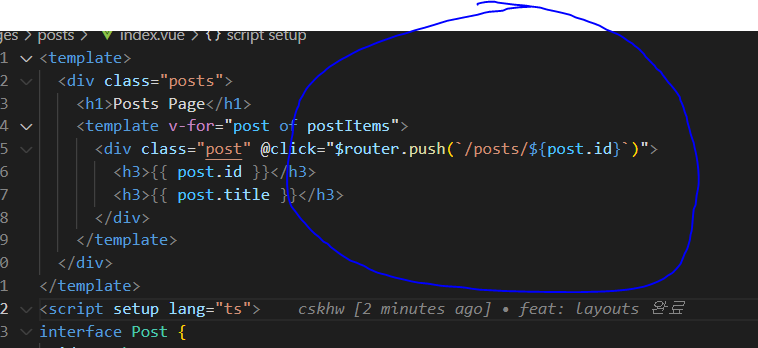
- router에서 url을 통해 id값을 전달하고 있는 것을 확인할 수 있습니다!
- 그런데 왜 id 값만 가져오고 게시글의 값은 안가져와? 라는 의문이 드실거에요. 이것은 나중에 스토어에 대해서 배워보면서 풀어 나가봅시다.
pages 끝!
pages는 홈페이지의 각 페이지들이 저장됩니다. Nuxt에서 자동으로 라우팅 설정을 해주기 때문에 따로 라우팅 설정을 안해도 되는 간편함이 있습니다.
- nuxt에서 sass, scss를 사용하기 위해서 아래 명령어를 입력해줍니다.
npm install sass
- app.vue에 아래 코드를 넣어줍니다.
<template>
<nav>
<NuxtLink to="/">home</NuxtLink>
<NuxtLink to="posts">posts</NuxtLink>
<NuxtLink to="profile">profile</NuxtLink>
</nav>
<NuxtPage />
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped>
nav {
& > a {
text-decoration: none;
color: green;
&:hover {
color: blueviolet;
}
}
display: flex;
gap: 8px;
}
</style>
- NuxtLink는 라우트 해주는 컴포넌트로 to안에 원하는 경로를 입력합니다.
- script옆의 setup은 vue3에서 제공해주는 setup스크립트를 사용한다는 의미입니다. docs: https://vuejs.org/api/sfc-script-setup.html
- vue3의 setup 함수를 스크립트 전체에 적용하며 코드의 가독성과 모듈성을 매우 높여줍니다.
- style 옆의 scoped는 현재 컴포넌트에만 css를 적용한다는 의미입니다. 자세한 내용은 나중에 봅시다.
- pages 폴더 만들기 > 안에 index.vue 파일 만들기 > 아래 내용 넣기
<template>
<div class="home">
<h1>Index Page</h1>
</div>
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped></style>
- 아래와 같은 화면을 볼 수 있습니다.
- pages 폴더 안에 profile.vue 만들기 > 아래 내용 넣기
<template>
<div class="profile">
<h1>Profile Page</h1>
<div class="profile-img"></div>
<h1>넉스트</h1>
<h2>나는 넉스트 마스터</h2>
</div>
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped>
.profile {
.profile-img {
width: 50px;
height: 50px;
border-radius: 50%;
background-color: gray;
}
}
</style>
- 이제 홈으로 가서 네비게이션의 profile 버튼을 눌러보세요. /posts/profile로 이동하는 것을 볼 수있습니다. Nuxt에서는 폴더 구조에 따라서 url을 자동으로 잡아줍니다. 겁나 편해요.
- pages 폴더 안에 posts 폴더 만들기 > 안에 index.vue 파일 만들기 > 아래 내용 넣기
<template>
<div class="posts">
<h1>Posts Page</h1>
<template v-for="post of postItems">
<div class="post" @click="$router.push(`/posts/${post.id}`)">
<h3>{{ post.id }}</h3>
<h3>{{ post.title }}</h3>
</div>
</template>
</div>
</template>
<script setup lang="ts">
interface Post {
id: number;
author: string;
title: string;
contents: string;
}
const postItems = ref<Post[]>([
{
id: 1,
author: "foo",
title: "첫번째 게시글!",
contents: "첫번째 게시글 내용!",
},
{
id: 2,
author: "foo",
title: "세상에서 제일 귀여운 고양이",
contents: "구라얌",
},
]);
</script>
<style lang="scss" scoped>
.post {
display: flex;
gap: 8px;
align-items: center;
cursor: pointer;
&:hover {
opacity: 0.8;
}
}
</style>
- posts폴더 안에 [id].vue 폴더 만들기 > 아래 내용 넣기
<template>
<div class="post">
<h1>Post</h1>
<NuxtLink to="/posts">뒤로 가기</NuxtLink>
<h2>id: {{ $route.params.id }}</h2>
</div>
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped></style>
- 결과적으로 이런 폴더 구조가 만들어집니다.
- 이제 /posts에 접속하면 포스트 리스트들을 볼 수 있고 클릭하면 각 포스트로 이동하는 것을 볼 수 있습니다. !: 혹시 보이지 않는다면 새로 고침 혹은 터미널에서 컨트롤 + c 연타 후 npm run dev를 실행해주세요.
- 무슨 원리일까요? posts 아래에 [id].vue파일을 보면 [] 안에 id라는 문자가 들어 있습니다. 이 아이디 어디서 많이 보지 않았나요?
- router에서 url을 통해 id값을 전달하고 있는 것을 확인할 수 있습니다!
- 그런데 왜 id 값만 가져오고 게시글의 값은 안가져와? 라는 의문이 드실거에요. 이것은 나중에 스토어에 대해서 배워보면서 풀어 나가봅시다.
pages 끝!
pages는 홈페이지의 각 페이지들이 저장됩니다. Nuxt에서 자동으로 라우팅 설정을 해주기 때문에 따로 라우팅 설정을 안해도 되는 간편함이 있습니다.
- nuxt에서 sass, scss를 사용하기 위해서 아래 명령어를 입력해줍니다.
npm install sass
- app.vue에 아래 코드를 넣어줍니다.
<template>
<nav>
<NuxtLink to="/">home</NuxtLink>
<NuxtLink to="posts">posts</NuxtLink>
<NuxtLink to="profile">profile</NuxtLink>
</nav>
<NuxtPage />
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped>
nav {
& > a {
text-decoration: none;
color: green;
&:hover {
color: blueviolet;
}
}
display: flex;
gap: 8px;
}
</style>
- NuxtLink는 라우트 해주는 컴포넌트로 to안에 원하는 경로를 입력합니다.
- script옆의 setup은 vue3에서 제공해주는 setup스크립트를 사용한다는 의미입니다. docs: https://vuejs.org/api/sfc-script-setup.html
- vue3의 setup 함수를 스크립트 전체에 적용하며 코드의 가독성과 모듈성을 매우 높여줍니다.
- style 옆의 scoped는 현재 컴포넌트에만 css를 적용한다는 의미입니다. 자세한 내용은 나중에 봅시다.
- pages 폴더 만들기 > 안에 index.vue 파일 만들기 > 아래 내용 넣기
<template>
<div class="home">
<h1>Index Page</h1>
</div>
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped></style>
- 아래와 같은 화면을 볼 수 있습니다.
- pages 폴더 안에 profile.vue 만들기 > 아래 내용 넣기
<template>
<div class="profile">
<h1>Profile Page</h1>
<div class="profile-img"></div>
<h1>넉스트</h1>
<h2>나는 넉스트 마스터</h2>
</div>
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped>
.profile {
.profile-img {
width: 50px;
height: 50px;
border-radius: 50%;
background-color: gray;
}
}
</style>
- 이제 홈으로 가서 네비게이션의 profile 버튼을 눌러보세요. /posts/profile로 이동하는 것을 볼 수있습니다. Nuxt에서는 폴더 구조에 따라서 url을 자동으로 잡아줍니다. 겁나 편해요.
- pages 폴더 안에 posts 폴더 만들기 > 안에 index.vue 파일 만들기 > 아래 내용 넣기
<template>
<div class="posts">
<h1>Posts Page</h1>
<template v-for="post of postItems">
<div class="post" @click="$router.push(`/posts/${post.id}`)">
<h3>{{ post.id }}</h3>
<h3>{{ post.title }}</h3>
</div>
</template>
</div>
</template>
<script setup lang="ts">
interface Post {
id: number;
author: string;
title: string;
contents: string;
}
const postItems = ref<Post[]>([
{
id: 1,
author: "foo",
title: "첫번째 게시글!",
contents: "첫번째 게시글 내용!",
},
{
id: 2,
author: "foo",
title: "세상에서 제일 귀여운 고양이",
contents: "구라얌",
},
]);
</script>
<style lang="scss" scoped>
.post {
display: flex;
gap: 8px;
align-items: center;
cursor: pointer;
&:hover {
opacity: 0.8;
}
}
</style>
- posts폴더 안에 [id].vue 폴더 만들기 > 아래 내용 넣기
<template>
<div class="post">
<h1>Post</h1>
<NuxtLink to="/posts">뒤로 가기</NuxtLink>
<h2>id: {{ $route.params.id }}</h2>
</div>
</template>
<script setup lang="ts"></script>
<style lang="scss" scoped></style>
- 결과적으로 이런 폴더 구조가 만들어집니다.
- 이제 /posts에 접속하면 포스트 리스트들을 볼 수 있고 클릭하면 각 포스트로 이동하는 것을 볼 수 있습니다. !: 혹시 보이지 않는다면 새로 고침 혹은 터미널에서 컨트롤 + c 연타 후 npm run dev를 실행해주세요.
- 무슨 원리일까요? posts 아래에 [id].vue파일을 보면 [] 안에 id라는 문자가 들어 있습니다. 이 아이디 어디서 많이 보지 않았나요?
- router에서 url을 통해 id값을 전달하고 있는 것을 확인할 수 있습니다!
- 그런데 왜 id 값만 가져오고 게시글의 값은 안가져와? 라는 의문이 드실거에요. 이것은 나중에 스토어에 대해서 배워보면서 풀어 나가봅시다.
pages 끝!
728x90
반응형